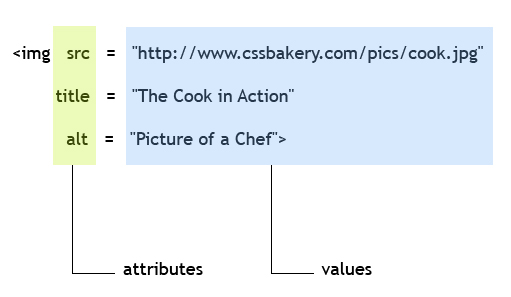
It has 3 attributes that are listed as src, title, and alt. Since all attributes have values, src gets "cook.jpg" with its full path, title has "cook in action" and alt has "picture of a chef" as their values. Even if you have to assign an empty string, a value has to be present for any attribute name that is included in your element. Attribute values are always surrounded by double quotes. We'll be hooking into these attributes in different ways, by writing special CSS selectors called attribute selectors. We are going to working on the style sheet but do keep in mind that the elements and their attributes come from the markup and not your CSS.
BASIC ATTRIBUTE SELECTOR
There are several different types of attribute selectors in CSS. The most elementary form of it acts on the mere existence of an attribute name. Even though all attributes will have values, the selector itself does not concern itself with what's assigned to the attribute. As the CSS standard says, this type of selector will match when the element sets the attribute, whatever the value of the attribute.
Let's see about writing a simple attribute selector for an img element. In standards compliant CSS, we are supposed to always include the alt attribute when we use the img element. If you wanted to quickly see which img elements included the alt attribute, you could write:
img[alt] { border: 1px dotted black; }
where the attribute selector "img[alt]" will select all the img elements with an alt attribute, and we'll draw a single pixel dotted black border around them. It doesn't matter what the alt attribute is set to - as long as there's an alt within the img element, it'll be matched by this selector. This is a simple attribute selector because it acts only on the presence of a specified attribute.
"class" is another commonly used attribute in CSS so to change the look of span elements with classes, you could write:
span[class] { font-weight: bold; }
Our example selector together with the markup:
This is a new song named <span class="cd">Tangerine Dreams</span> which is the soundtrack of
the movie <span class="movies">Summer Splash</span>
would style our text as follows:
This is a new song named Tangerine Dreams which is the soundtrack of the movie Summer Splash.
Both of these span elements will be matched by our selector based on them having the class attribute regardless of their differing values for the specified attribute. Simple attribute selectors select elements based on them having a certain attribute or multiple attributes. If we reconsider the img element above to make it a multiple attribute selector, you could write a selector that will target the img elements which have both the alt and title attributes:
img[alt][title] { border: 1px solid black; }
will select:
<img src="images/thecook.jpg" title="The Cook in Action" alt="Picture of a Chef" />
but not:
<img src="images/thecook.jpg" alt="Picture of a Chef" />
The basic attribute selector syntax has the attribute name contained in square brackets. When you need to specify more than one attribute, you list them back to back, with each attribute enclosed in its own square brackets. The attribute selector is followed by declarations in curly braces just like any other selector.
The next kind of attribute selector goes one step further by requiring a match of values: An attribute value selector will match when the element's attribute value is exactly the value that you specify in your selector. Let's look at that in more detail.
VALUE ATTRIBUTE SELECTORS
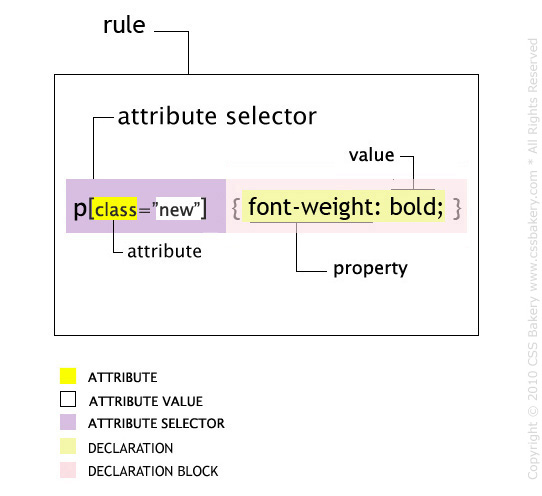
el[att="val"] --> matches when the element's "att" attribute value is exactly "val".
Value attribute selectors go one step further than the basic attribute selector and look for an exact match of its attribute value in the elements. This type of selector will match any element "el" with an "att" attribute whose attribute value is exactly "val". In the following example, we have two attribute selectors that match based on value, boldfacing one span element and italicizing the other.
First our markup,
This is a new song named <span class="cd">Tangerine Dreams</span> which is the soundtrack of
the movie <span class="movies">Summer Splash</span>
Example selectors,
span[class="cd"] { font-weight: bold; }
span[class="movies"] { font-style: italic; }
will have the following effect on the text:
This is a new song named Tangerine Dreams which is the soundtrack of the movie Summer Splash.
The value attribute selectors are different than our basic attribute selector where we only had to specify the attribute name ("class" in the previous example). span[class] {font-weight: bold;} made both of the span element strings bold. With a value attribute selector, we were able to pick and choose between different classes. Instead of more sweeping changes, we targeted the right class for our actions which is how value attribute selectors can help you, too.
PARTIAL VALUE ATTRIBUTE SELECTOR
These selectors are similar to value attribute selectors but instead of having an exact match, you can select elements based on a partial match of the attribute value. There are 4 kinds of partial value attribute selectors, each of which use a different symbol. These are the tilde ~, the carrot ^, the asterisk *, and the dollar $ signs, all of which come right before the equal sign in your selector.
With the tilde ~ character, you can write a selector which will match an element with an attribute whose value is a white space separated list of words, one of which matches your chosen attribute value. To match one of the attributes of the multi-class p element and select it,
<p class="connection fontdesign textstyle">The text for the new book ......</p>
you would write:
p[class~="fontdesign"] {......}
The remaining partial value selectors are known as substring matching attribute selectors and were added to CSS after CSS2 (in a subsequent module).
el[att^="val"] Matches any element "el" with an "att" attribute that begins with "val".
el[att$="val"] Matches any element "el" with an "att" attribute that ends with "val".
el[att*="val"] Matches any element "el" with an "att" attribute that contains the substring "val".
A partial value attribute selector with the carrot ^ sign will allow you to match attribute values that begin with your specified attribute value and either are equal to or longer values than your specified attribute value.
A partial value attribute selector with the dollar $ sign will select any element whose attribute value ends with your specified attribute value.
A partial value attribute selector with an * asterisk will select any element whose attribute value contains your specified attribute value somewhere in it. As long as your attribute value is a substring of its attribute value, that element will be selected.
PARTICULAR ATTRIBUTE SELECTOR
el[att|="val"] --> matches any element "el" with an "att" attribute that is either equal to "val", or begins with "val", immediately followed by a dash and continuing with any other characters.
The syntax has brackets and a "|=" sign and is used to select hyphenated elements. It is especially helpful targeting specific language declarations, where you might have hyphenated attributes in an XHTML element.
p[class|="stylized_olho"] { text-indent: 86px; padding-top: 60px; }
will match all occurrences of the element p with a "class" attribute where "stylized_olho" is the beginning of the value string for the class name. So values such as "stylized_olho-A", "stylized_olho-letter", "stylized_olho-a", or "stylized_olho-b" are valid matches for the selector.
If you are wondering what purpose this type of selector serves, I had twenty six (26) different but similar p elements that all needed the same CSS rules applied. I could have written 26 of them one by one but instead I took the attribute selector shortcut to tell CSS to match all p elements that have different attribute names (can think of these as variable names) which start with "stylized_olho-". I've had this type of selector in my drop cap code and did not encounter compatibility problems in either IE7.0 or Firefox. Test your code in different browsers/versions especially when you use substring matching.
Post a Comment
Note: Only a member of this blog may post a comment.