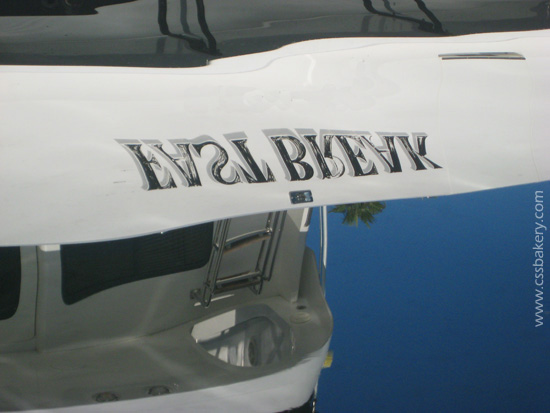
Having promised myself and you a post a day in October, I have some catching up to do on this Halloween day. We'll go back to the PHP code that we were working on for the mail order company mimicking the J.Crew site. I'll keep posting under this title as I get chunks of the code finished.
To make this example more like a real online shopping site I've designed a three column layout, and a menu that will be common to all the pages of the site. In the layout, only the middle column content will change from page to page. We want to accomplish this without having to duplicate site content in multiple files. There are many ways to do this in PHP. I'll take a simple approach of just factoring out all the common parts into separate files, which will then be included in various files that are specific to the middle column content.
Here's a picture of what the file structure will look like:
header.php holds one piece of common code. This is where we put the menu that goes into column one of every page. The other common code goes into footer.php. Here we have some common Javascript along with the actual footer content which lists some common site links.
The files showitems.php, and shoes_complete.php will each provide unique content for the middle column. In showitems.php this will be a list of all the products in the database (i.e. all shoes listed, with a clickable link to get more detail on a specific shoe). shoes_complete.php will show details on a single shoe, given the shoe's item number, passed as a parameter in the URL.
So now, with the addition of showitems.php you no longer have to type in a URL with an item number to see the details of particular shoe. Instead, the code in showitems.php generates hyperlinks with the complete URL already filled in with the itemnumber for the corresponding shoe. When you click the link, the item number is passed to shoes_complete.php in the query parameter portion of the URL.
In this post I want to extend the previous PHP/MySQL examples, by generating a page that shows a list of all the shoes in the database. This will include a small image of the shoe, the price, and the name of the shoe with a hyperlink to get more detail for ordering the shoe.
With a small change to the MySQL queries from previous posts, we can get all records from the shoe_primary_data table (before we were getting only a specific record via the where clause like this: where itemnum='$itemnum'). Here, we simply leave the where clause off of the query, and the database will default to returning all records:
// make a connection to the mysql database server $db = mysqli_connect("womenshoes.db.6787492.hostedresource.com","womenshoes", "thisIsyourPassword", "womenshoes"); if ($db) { //SHOE_PRIMARY_DATA TABLE //building a query string to send to the database, this will be the search string //Give me all the records, with all the fields (star) from the shoe_primary_data table $query = "SELECT * FROM shoe_primary_data";
Now that we've got all records from shoe_primary_data, we will loop through them and generate a HTML list of items, like this:
<div id="container"> <ul id="shoeList"> <?php while ($row=mysqli_fetch_array($result,MYSQLI_ASSOC)): ?> <li> <div> <img style="width:80px;" src="<?=$row['imagepath']?>"> <div><a href="shoes_complete.php?itemnum=<?=$row['itemnum']?>"> <?=$row['itemname']?></a></div> <div><?php printf("$%01.2f",$row['price'])?> </div> </div> </li> <?php endwhile; ?> </ul> </div>
What we have here is snippets of PHP interspersed with HTML. The PHP in this case, loops through all the records retrieved from the query, pulling each record into an associative array. For each record we generate a list, where each item in the list contains: (a) a small image of the product, (b) the name of the product as a hyperlink that takes you to more detail on the product, and (c) the price of the product.
The main thing to notice from this code is the structure of the while loop, where the call to mysqli_fetch_array() returns a result into the $row variable. If there are no more records mysqli_fetch_array returns false, and we drop out of the loop. Otherwise, we have the associative array for the current record sitting in the $row variable.
The rest is straightforward HTML. To style the resulting list we use the following CSS:
/* force vertical scroll bar to eliminate layout 'jumps' between pages */ html { overflow-y: scroll; } #container { width: 620px; /*padding-bottom: 400px;*/ } #container ul li { display: block; padding-left: 10px; width: 8em; text-align: center; float: left; list-style-type: none; } #container ul li img { display: block; float: none; list-style-type: none; margin: 5px; } #container ul li div { margin: 10px; }
1 comments:
I almost fell out of my chair laughing when I heard this song. This is every bit as good as Saturday Night Live's "not a witch" ad a few weeks ago.
Now, back to Cufon...
Post a Comment
Note: Only a member of this blog may post a comment.