Hi there!
I'm working on a form. On my form tag, the method attribute is set to 'post' and the action attribute points to a php file on my server. Data entered in forms makes its way to the web server as follows: When you submit the form, the Browser constructs a message in memory that contains all the field names and values from the form. The format of this message is dictated by the HTTP (RFC 2616) and HTML standards . In the usual case where the form tag includes "method='post'", the message will be a HTTP POST message. The browser builds the POST message according to the rules in RFC 2616, and includes all the fields and data on the form. The Browser then sends the message up to the server over a TCP connection.
When the web server software (Apache for example) receives the HTTP POST message, it passes it to the PHP interpreter which uses it to construct the $_POST superglobal variable to hold the values submitted in the form. Your PHP script then has access to what you entered on the form by just looking in the $_POST array.
Mail order pages contain good examples of forms. Take a look at this J.Crew page for a pair of shoes. We are going to create a similar page. First the markup:
<img src="images/jimmychoos.jpg" alt="Jimmy Choo Sandals" /> <div class="itemorder"> <h2>Strappy Evening Sandals</h2> <p>$275.00</p> <p> Elegance and pain defined. Classic special-occasion heel crafted in metallic or suede leather. Cross-over straps at toe and double ankle strap with gold metal self-fastening oval buckle. Suede lining and leather sole. 3 1/2" self-covered high heels that are sure hell to walk in. Made in the USA. Catalog/cssbakery.com only. </p> <form action="images/cbmailorder.php" method="POST"> <p>select size <select name="size"> <option value="5 H">5 H</option> <option value="6">6</option> <option value="6 H">6 H</option> <option value="7" selected="1">7</option> <option value="7 H">7 H</option> <option value="8">8</option> <option value="8 H">8 H</option> <option value="9">9</option> <option value="9 H">9 H</option> <option value="10">10</option> </select> </p> <p>select width <select name="width"> <option value="N">N</option> <option value="B" selected="1">B</option> <option value="W">W</option> </select> </p> <p>select color <select name="color"> <option value="gold">gold</option> <option value="platinum" selected="1">platinum</option> <option value="black suede">black suede</option> <option value="navy suede">navy</option> </select> </p> <p> <input type="text" name="quantity" value="" size="3"/> quantity </p> <div class="formrow"> <input type="image" src="images/blacksubmit.gif" name="" value="ADD TO BAG" /> </div> <p class="pa"> Need help updating your wardrobe? Looking for that perfect piece? Make an appointment with Magnolia, our personal shopping expert, at magnolia@cssbakery.com </p> </form> </div>
The CSS styles:
.formrow { margin: 15px; } .itemorder { float: left; margin: 30px; width: 250px; } img { float: left; padding-top: 26px; padding-left: 10px; } .itemorder p, input, select { color: #333333; font-family: verdana, geneva, arial; font-size: 10px; } .itemorder h2 { color:#333333; font-family:verdana,geneva,arial; font-size:14px; font-weight:bold; } form p { border-bottom: 1px solid #e0e0e0; padding-bottom: 7px; } .pa { border: none; }
Our Jimmy Choo page below is nearly identical to the J. Crew page:
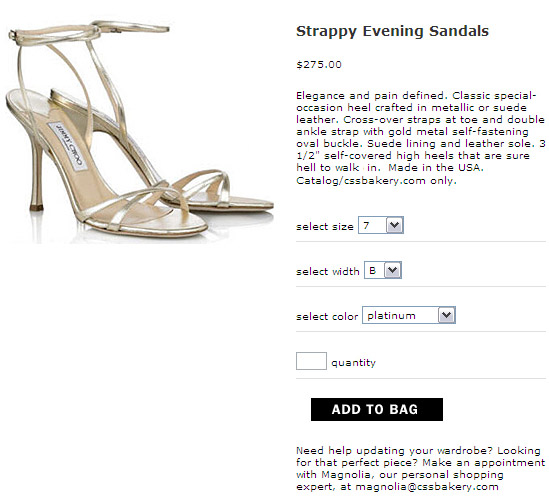
It's gotten really late here. I have to floss and brush while I still can. Tomorrow evening I'll straighten out this post, and we will write our PHP file and learn how to upload files to your own server at Godaddy.com.
Good night.
PHP FILE FOR THE MAIL ORDER COMPANY
6:30PM Tuesday on October 5th, The sun is going down in orange flames and I'm back. I want to first show you what the shoe page looks like without any CSS:
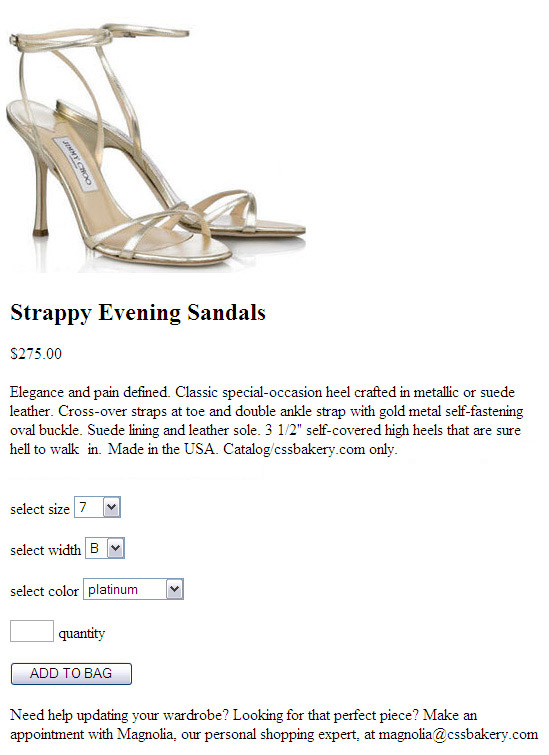
Last night in my hurry, I did not assign any names to form elements. We have size, color, width and quantity in our form. I'm going to create a PHP file named cbmailorder.php which is what the action attribute is set to in our form.
<form action="cbmailorder.php" method="POST">
Let's start with simple PHP and echo back the data collected from the end user. Variables in PHP start with a $ sign so:
<?php $size = $_POST['size']; $width = $_POST['width']; $color = $_POST['color']; $quantity = $_POST['quantity']; echo $size; echo $width; echo $color; echo $quantity; echo '<br />'; echo 'Thank you for shopping with CB Outlet<br />'; echo 'We will be shipping your '.$size.$width. ' shoes in ' . $color . ' tomorrow<br />'; echo 'You should receive your '. $quantity . ' pair of shoes in 4 business days<br />'; ?>
Note the use of the PHP echo command, which sends a string of data down to the browser. You can have PHP send down entire HTML documents this way, but there is another way that mixes native HMTL with PHP. Anything in a PHP file that isn't enclosed within a tag is sent straight through to the browser. So the last part of the code above that echo's out the confirmation message can be re-written as follows:
<p>Thank you for shopping with CB Outlet</p> <p>We will be shipping your <?php echo $size;?><?php echo $width?> shoes in <?php echo $color;?> tomorrow</p> <p>You should receive your <?php echo $quantity;?> pair of shoes in 4 business days</p>
This approach mixes in little snippets of PHP code with the HTML, whenever we need to do something like output a variable. When echoing variable like this, there is an even more compact way to write the code:
<p>Thank you for shopping with CB Outlet</p> <p>We will be shipping your <?=$size?><?=$width?> shoes in <?=$color?> tomorrow</p> <p>You should receive your <?=$quantity?> pair of shoes in 4 business days</p>
This method of getting PHP to echo a variable (<?=$theVariable?>) is called PHP short tags. The PHP documentation says this is "less portable" than the full length tags, and therefore "generally not recommended". However, many programmers use the short tags. The only issue you'll have is if you run into a Web hosting provider that has their php.ini settings configured to turn off short tags. If you have access to php.ini then you can just turn it on. I'm about to find out whether GoDaddy shared hosting has short tags turned on or not.
GODADDY SHARED HOSTING
Wednesday October 6, 9:45PM. They said it was record rain fall for today. I'm back to finalize the post.
Signing up with GoDaddy is easy. I already had one account with them for registering domain names. The new account I set up was for the web hosting plan to show you how that is done. They offer three different types of hosting plans, I chose the first option - Grid hosting with Linux - which has everything that we need for this simple project.
Once you get the account ready, you can start uploading your files to your new server. There are many ways to do this including using FileZilla, or from Eclipse. For now I just have a couple of files to transfer so I'm going to use Godaddy file transfer from within their File Manager.
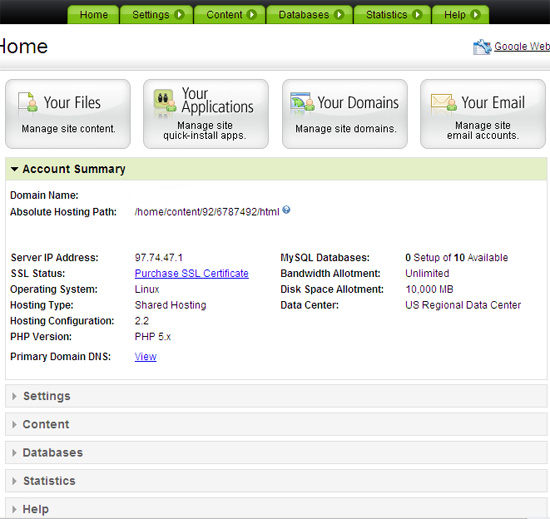
Under the Hosting Control Center, click on "Your Files" option. I created a new directory called "mailorder". Click on the new directory which should take you to an empty folder. Browse to upload your files. I uploaded four files, mailorder.html, cbmailorder.php, jimmychoos.jpg, and blacksubmit.gif.
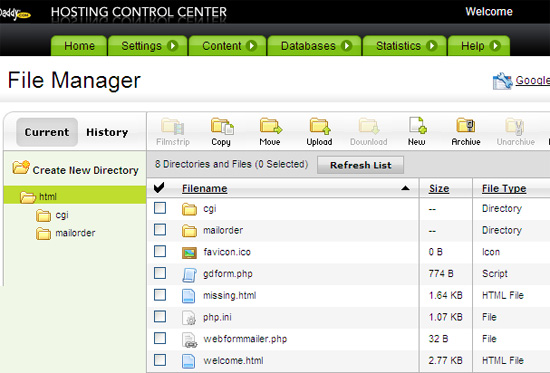
After uploading your files, if you want to change something, there's a way to edit directly on the server. If you click on the checkbox next to the filename, the "New" icon switches to "Edit" and you can take it from there. Don't forget to save once you are done. Godaddy site styling is clunky but it gets the job done without you having to reupload again.
So the files are there, in order to test our PHP, I opened a browser window and typed in http://www.exampledomain.com/mailorder/mailorder.html which prompted me with the item page for the shoes. You enter the data on the form: size, color, quantity and hit the submit button.
[Thursday October 7th, 6:30PM. More info on the PHP response]
Most programming books will fail to tell you the detailed technical details that are happening in the background during this time. A lot of events take place between an end-user's button click and the new page that appears on his screen. To him it's a computer miracle which is the way it should be, to most programmers it's a black box of events but there's a lot of work and juggling of processes behind the curtain. I'll try to describe it roughly.
Browser constructs an HTTP Post message containing the action from the form tag and all the fields and data that was entered on the form. Then opens a TCP connection to the server and transmits the POST message. The web server, example Apache, receives the POST message and sees that the action targets a PHP file in our case "cbmailorder.php". So it passes to the PHP interpreter the PHP file to be executed as well as the POST message contents. The PHP interpreter reads the POST message and builds the dollar sign underscore POST ($_POST) superglobal array. The PHP interpreter then executes your PHP script. Anything that you echo or anything that's outside of PHP tags is sent by the interpreter down to the browser. Anything that's HTML will be sent back to the browser without being interpreted, it just passes straight through but anything within the tags is considered part of PHP. The interpreter sends this data to the browser over the TCP connection that Apache had accepted from the browser (Or another way of saying it is the connection that was established between the browser and Apache).
The browser, your IE, Firefox or Safari, has a whole set of things to do. First it parses the HTML, CSS, and Javascript statements that are inline in the data it got back from the server. It then can begin downloading any external resources that are linked to in the HTML/CSS/Javascript, such as external CSS files, images, and external Javascript files. For these it builds additional HTTP GET messages and sends them up to the server over the already established TCP connection (however, if a resources is on a different server, it has to open a new TCP connections to that server). The browser can begin rendering the page before all resources are downloaded, but will complete all downloads before it says it is 'done' with the page. Once everything is downloaded and rendered, the browser is just processing events from the user such as mouse movements, key presses, clicks.
So after all that work, here's what you get on your screen as response to your submit. You can think of this as a simple debug with all the variable values checking out :
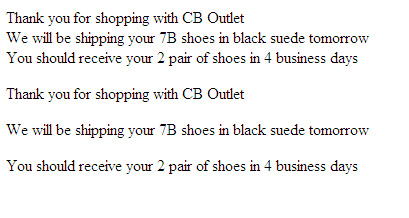
CONFIRMING SHORT TAG SUPPORT ON GODADDY
The following version of the PHP code adds three lines at the end to output the order confirmation message using PHP short tags. I left in the original echo statements as well for comparison. Note that the PHP ending tag (?>) occurs after these echo statements and before the newly added lines. That's because the newly added lines are HTML, not PHP. They do have some PHP statements embedded within them (using short tags, as described previously), but they are really HTML, so we don't want the PHP interpreter to see them as PHP code (which would produce a syntax error).
<?php $size = $_POST['size']; $width = $_POST['width']; $color = $_POST['color']; $quantity = $_POST['quantity']; echo 'Thank you for shopping with CB Outlet<br />'; echo 'We will be shipping your '.$size.$width. ' shoes in ' . $color . ' tomorrow<br />'; echo 'You should receive your '. $quantity . ' pair of shoes in 4 business days<br />'; ?> <p>Thank you for shopping with CB Outlet</p> <p>We will be shipping your <?=$size?><?=$width?> shoes in <?=$color?> tomorrow</p> <p>You should receive your <?=$quantity?> pair of shoes in 4 business days</p>
2 comments:
Awesome site you have here but I was wanting to know
if you knew of any user discussion forums
that cover the same topics talked about in this article?
I'd really like to be a part of group where I can get responses from other knowledgeable individuals that share the same interest. If you have any recommendations, please let me know. Bless you!
My homepage :: social bookmarking service
my webpage: social bookmark
There is Boston PHP which is a meetup that you can attend if you are in the New England area. For online reference, also Zend, and PEAR.
Post a Comment
Note: Only a member of this blog may post a comment.