Finally at the computer this Sunday October 10th at 5:45 pm, I am two days behind in my blog posts. We'll finish with Bob Parsons - he is the Godaddy man - in the next post, right now I want to talk about using cookies and sessions to store a location for a Google Maps Application. I am writing here under Friday's post because I want to avoid having lone image posts whenever I can.
I'm starting to work with the Google maps API, and I've got a map that displays some local information, along with a field that allows you to enter a new location you are interested in. So you can enter a city & state, or a zip code. Send this location up to a server, get back some information on the new location, and then re-display the map.
That works fine, but suppose you want to remember the latest location the user was interested in, so that next time they visit the site you can take them back to that location automatically? That's where cookies comes in. Cookies are little bits of data you can store on the user's browser. After your server has sent the cookie down to the browser, then the browser will send that cookie back to the server for each new request.
So, in PHP you can have the server send down a cookie like this:
setcookie("location", "San Francisco, CA", time()+3600*365);
The parameters passed to setcookie() are
- the name of the cookie
- the value to store
- and when the cookie should expire (in this case it will expire one year later - the current time + number of seconds in a day (3600) times number of days in a year (365))
There are actually more optional parameters that you can read about in the PHP manual, but those three are the common ones.
One thing you do have to make sure of when using cookies is that you send the cookie to the browser before you send anything else. So you can't send a cookie after you've already started sending down HTML.
Now that the cookie is set, the browser will send it back to the server, and from PHP you can read it like this:
$location = $_COOKIE["location"];
Now suppose that your site is getting more complex and you find you have a lot of different cookies to store various bits of data. Browsers have a limit on how many they will accept from a single web site, so you might take a different approach where you store the user's data in a database table, then send down a single cookie that identifies the record in the database. This is know as a "session", and the value you put in the cookie is the "session id". The database table has a key that equals the session id, so that when the browser sends back the cookie, you grab the session id from the cookie, read the record from the database, and you've got all the data - using only a single cookie! This also has the advantage that you send just a little data back and forth with the browser (i.e. the session id), but you can store a lot of data in the database record.
There are other things you need to do to properly manage sessions (things like making sure you don't have a bunch of old sessions laying around that are no good to anyone). But if you use an application framework it likely has a session mechanism built in. In my case I am using the Code Igniter framework, and it takes care of session management. So here's what I did to store the user's location:
$location = $this->uri->segment(4); if (empty($location)) { $location = $this->session->userdata('location'); } else { $this->session->set_userdata('location', $location); }
First I grab the location from the URL, then check if what I got is empty. If it is then it means the user didn't send me any location, so I read it from the value stored in the session. Otherwise, in the else case, the user has sent me a location in the URL, so I store that into the session. Later in my database model I use the location as part of a query. That code does have to handle the case where the location is not set (i.e. the case where the user didn't send a location in the URL, and there is no location stored in the session). What I do there is just pick a default location in the middle of the US and make the map zoom out to see all of North America (similar to what you see when you go to maps.google.com).
So hopefully that gives you a little insight into what cookies and sessions are, and how they can be useful. Here's a screenshot of a Google Map that I programmed:
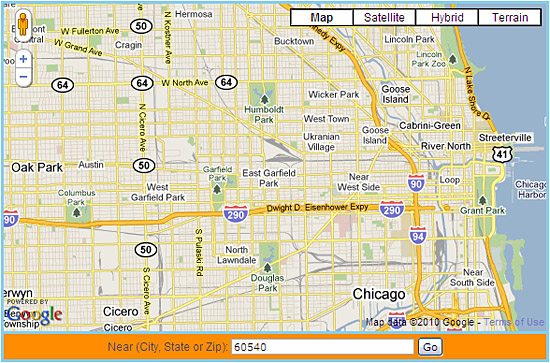
Post a Comment
Note: Only a member of this blog may post a comment.